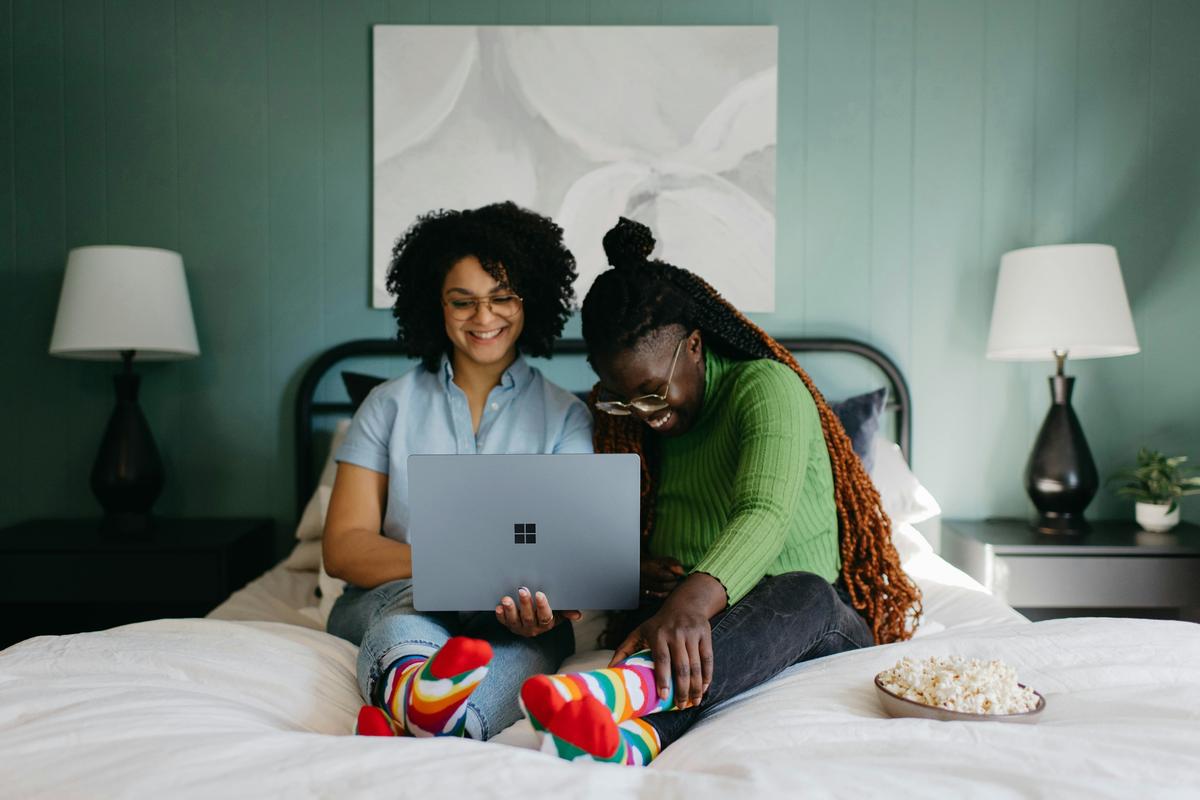
GitHub
Understanding useMemo and useCallback
Understanding useMemo
and useCallback
In the world of React, performance optimization is crucial to ensure that our applications remain fast and responsive. One of the most effective ways to achieve this is by using React hooks such as useMemo
and useCallback
.
What are useMemo
and useCallback
?
useMemo
and useCallback
are two separate hooks that serve a similar purpose: to memoize (remember) values or functions. The main difference between the two lies in their usage and scope.
useMemo
: Memoizing Values
import { useState, useMemo } from 'react';
function App() {
const [count, setCount] = useState(0);
const doubleCount = useMemo(() => {
return count * 2;
}, [count]);
return (
<div>
Count: {count}
Double Count: {doubleCount}
</div>
);
}
As shown above, useMemo
returns a memorized value that's only recalculated when its dependencies change. In this case, if the count changes, the doubleCount will be recalculated accordingly.
useCallback
: Memoizing Functions
Functions can also be memoized using useCallback
. This hook is particularly useful when we need to preserve the reference of a function throughout the component's lifetime.
Here's an example:
import { useState, useCallback } from 'react';
function App() {
const [count, setCount] = useState(0);
const handleIncrement = useCallback(() => {
setCount(count + 1);
}, [count]);
return (
<div>
Count: {count}
<br>
<button onClick={handleIncrement}>Increment</button>
</div>
);
}
useCallback
returns a memorized function that's only recreated when its dependencies change. This ensures that the function remains the same throughout the component's lifetime, which can be useful in scenarios where we need to pass a function as a prop or use it as an event handler.
Best Practices for Using useMemo
and useCallback
- Use dependencies wisely: Make sure to include all the dependencies that affect the memoized value or function. If not, you might end up with a memoized value or function that's not updated when it should be.
- Avoid unnecessary re-renders: By memoizing values or functions, we can reduce the number of re-renders and improve performance. However, if we don't include all the necessary dependencies, we might end up with stale data or incorrect function references.
- Test and verify: Always test and verify that your memoized values or functions are working as expected. This includes checking for any unexpected re-renders or updates.
Conclusion
In conclusion, useMemo
and useCallback
are powerful React hooks that can help us improve the performance and efficiency of our applications. By understanding how to use these hooks effectively, we can create faster and more responsive user interfaces that provide a better user experience.
Remember to use dependencies wisely, avoid unnecessary re-renders, and test and verify your code to ensure that it's working as expected.