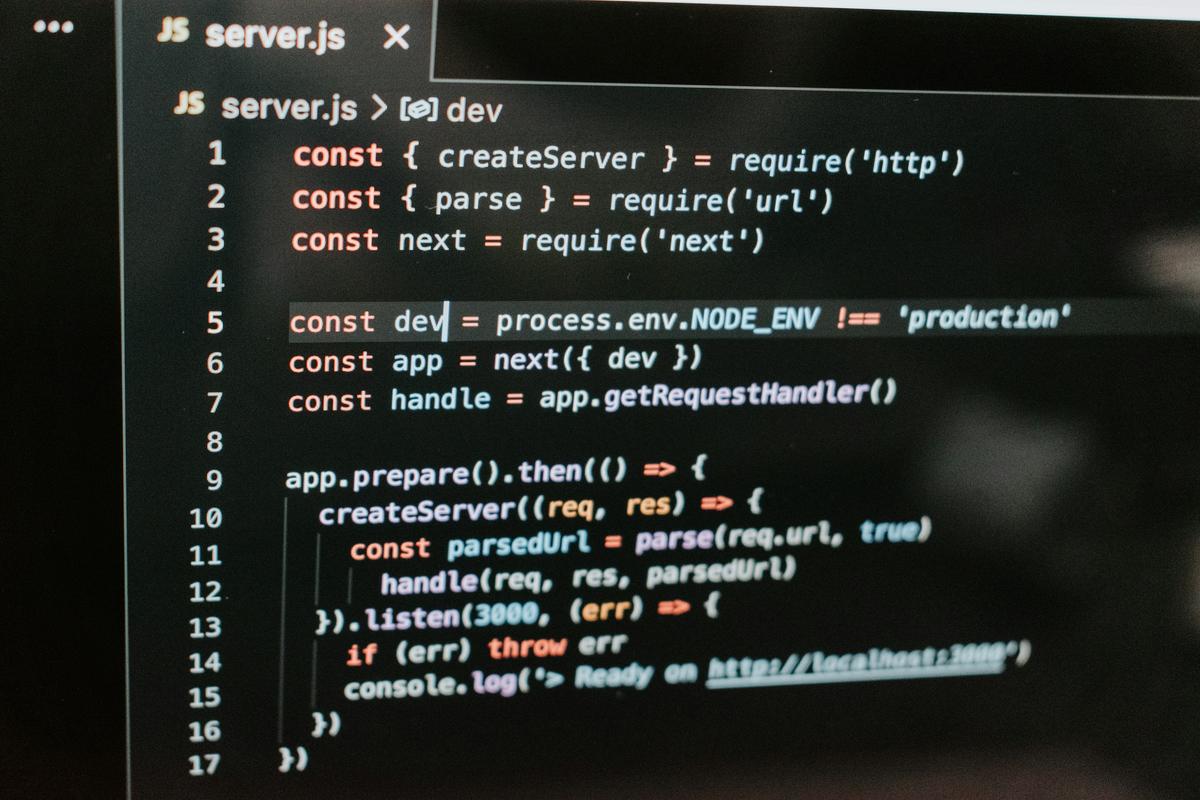
GitHub
how to use prisma with nextjs ?
How to use Prisma with Next.js?
Prisma is a popular ORM (Object-Relational Mapping) tool for Node.js applications that provides a flexible and powerful way to interact with databases. Next.js is a React-based framework for building server-side rendered (SSR) and statically generated websites. Combining Prisma with Next.js can help you build robust, scalable, and maintainable applications with ease. In this article, we'll explore the steps to integrate Prisma with Next.js.
Prerequisites
To get started, make sure you have the following:
Node.js installed on your system
Prisma installed as a dependency in your project
a Next.js project set up
a database setup (e.g., PostgreSQL, MySQL, or SQLite)
Step 1: Create a Prisma Schema
The first step is to create a Prisma schema file (e.g., schema.prisma
) and define your database models. This file acts as a communication layer between your application and the database.
model User {
id String @id @default(cuid())
name String
email String @unique
}
This schema defines a simple User
model with three attributes: id
, name
, and email
. The @id
and @default(cuid())
annotations ensure that the id
field is automatically populated with a unique identifier.
Step 2: Generate Prisma Migrations
After defining your schema, you'll need to generate Prisma migrations. This process will create the necessary database tables based on your schema definitions.
npx prisma migrate dev
This command generates the initial migration file and creates the corresponding database tables.
Step 3: Connect to the Database
To connect to your database, create a Prisma Client instance and provide the necessary database connection details.
import { PrismaClient } from '@prisma/prisma-client';
const prisma = new PrismaClient();
async function main() {
const users = await prisma.user.findMany();
console.log(users);
}
main();
This code snippet creates a Prisma Client instance and uses it to fetch a list of users from the database.
Step 4: Integrate with Next.js
To integrate Prisma with Next.js, create a Next.js API route that uses the Prisma Client to interact with the database.
// pages/api/users.js
import { NextApiRequest, NextApiResponse } from 'next';
import { PrismaClient } from '@prisma/prisma-client';
const prisma = new PrismaClient();
export default async function handler(req: NextApiRequest, res: NextApiResponse) {
switch (req.method) {
case 'GET':
const users = await prisma.user.findMany();
return res.status(200).json(users);
case 'POST':
const { name, email } = req.body;
const user = await prisma.user.create({ data: { name, email } });
return res.status(201).json(user);
default:
return res.status(405).json({ message: 'Method not allowed' });
}
}
This API route handles GET and POST requests. For GET requests, it fetches a list of users from the database. For POST requests, it creates a new user in the database.
Conclusion
In this article, we've covered the steps to integrate Prisma with Next.js. From creating a Prisma schema to generating migrations and connecting to the database, we've explored the necessary procedures to get started with Prisma and Next.js. By combining these two powerful tools, you can build robust, scalable, and maintainable applications with ease. Whether you're building a simple blog or a complex e-commerce platform, Prisma and Next.js provide a solid foundation for your project.
Remember to always follow best practices for security and performance when using Prisma and Next.js. Stay tuned for more tutorials and articles on building powerful applications with Prisma and Next.js.
Tags: prisma
, nextjs
Category: b0432347-8c05-42fe-a886-c8a6c242c548