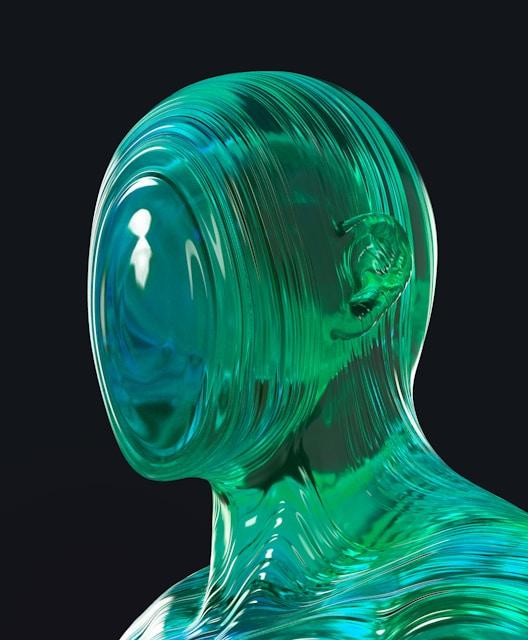
GitHub
beginner guide to build ai agent using llm
Beginner's Guide to Building an AI Agent using LLMl
As AI technology continues to advance, the need for AI agents has never been more pressing. With the integration of Large Language Models (LLMs) in AI development, the possibility of creating intelligent machines has become a reality. However, building an AI agent that can effectively interact with humans and complete tasks autonomously is a complex process that requires a solid understanding of AI principles, LLMs, and programming languages.
What is an LLM?
LLMs, or Large Language Models, are pre-trained AI models that can perform various natural language processing tasks, such as language translation, text generation, and sentiment analysis. They are trained on massive datasets of text and can generate human-like responses to specific inputs.
The Purpose of LLM in AI Agents
LLMs play a crucial role in AI agent development as they enable agents to understand and generate human language. This allows agents to process and respond to user queries, making them more effective in tasks such as customer service, language translation, and text summarization.
Building an AI Agent using LLM
Building an AI agent using LLM involves several steps, including:
Choosing the right LLM: With the increasing popularity of LLMs, there are many models to choose from. Popular LLMs include OpenAI's BART and T5, which are capable of generating high-quality text.
Preparing the dataset: The next step is to prepare a dataset for training the LLM. This can include manually annotating data or using pre-annotated datasets.
Training the LLM: Once the dataset is prepared, it's time to train the LLM using a programming language like Python.
Integrating the LLM with an AI agent: After training the LLM, it needs to be integrated with an AI agent framework, such as the OpenAI Gym or POMDP-Solver.
Testing and refining: The final step is to test the AI agent and refine its performance by tweaking the model or dataset.
Tools and Technologies
Building an AI agent using LLM requires a combination of programming languages, frameworks, and libraries. Some popular tools and technologies for AI agent development include:
Python: A popular programming language for AI development, Python is widely used for its simplicity and extensive libraries.
TensorFlow and PyTorch: Two popular deep learning frameworks used for training LLMs.
OpenAI Gym: A popular framework for training AI agents using reinforcement learning.
POMDP-Solver: A Python library for solving Partially Observable Markov Decision Processes (POMDPs).
Conclusion
Building an AI agent using LLM is a complex process that requires a solid understanding of AI principles, LLMs, and programming languages. By following the steps outlined in this guide and using the right tools and technologies, you can create an AI agent that is capable of completing tasks autonomously and interacting with humans effectively. Whether you're a beginner or an experienced AI developer, this guide aims to provide a comprehensive introduction to the process of building an AI agent using LLM.
# Importing necessary libraries
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# Loading dataset
dataset = pd.read_csv('dataset.csv')
# Preprocessing dataset
X = dataset.drop('target', axis=1)
y = dataset['target']
# Splitting dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Training Random Forest classifier on LLM
clf = RandomForestClassifier(n_estimators=100, random_state=42)
clf.fit(X_train, y_train)